As C programmers, we often navigate through the intricate web of strings, akin to untangling a complex knot. Understanding and utilizing the 10 essential string functions is akin to having a reliable set of tools to unravel these strings efficiently and effectively.
From manipulating and comparing strings to customizing their formats, these functions serve as the backbone of our string operations. Whether it's duplicating, concatenating, or searching for substrings, these functions are indispensable assets in our programming toolkit.
But what makes these functions truly essential? Let's explore their practical applications and the impact they have on our proficiency as C programmers.
String Copy and Duplicate
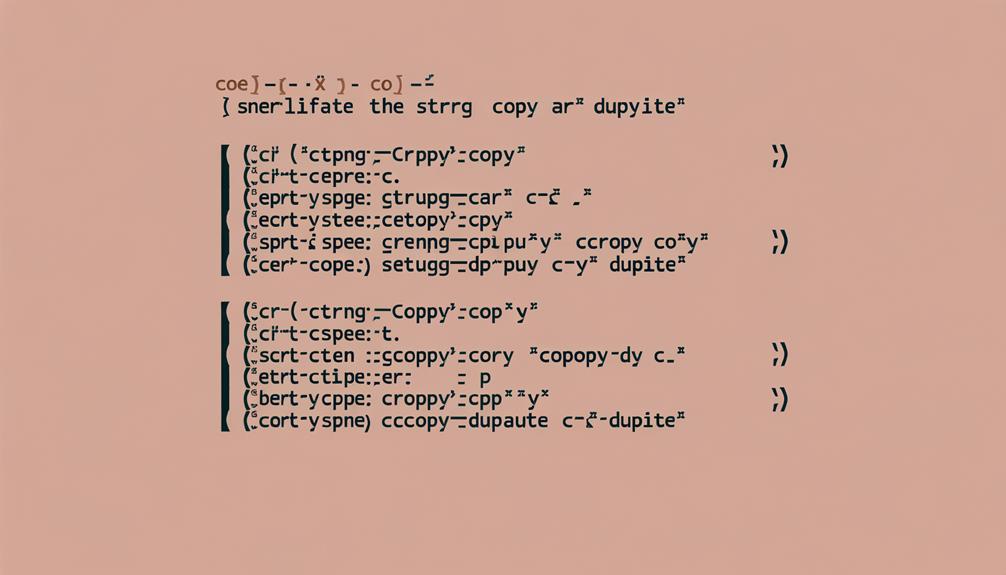
Strncpy() and strdup() are essential string functions in C programming, facilitating the copying and duplication of strings with precision and efficiency.
Strncpy() allows us to copy a specified number of characters from one string to another, ensuring accurate and controlled data transfer.
On the other hand, strdup() enables us to create a duplicate of a string, providing the freedom to manipulate the duplicated string independently.
These functions are liberating for programmers, offering the ability to work with strings in a more flexible and efficient manner.
Whether it's creating backups of string data or extracting specific portions for manipulation, Strncpy() and strdup() empower us to handle string operations with confidence and precision.
String Concatenate
String Concatenate serves as another essential string function in C programming. It allows for the efficient combination of multiple strings into one cohesive unit. This function is crucial for tasks such as creating dynamic messages, constructing file paths, and formatting output.
The strncat() function provides a way to concatenate a specified number of characters from the second string to the end of the first string. This feature is beneficial for efficiently merging strings without excessive memory allocation. It simplifies the process of building complex strings by appending only the necessary content.
String Compare
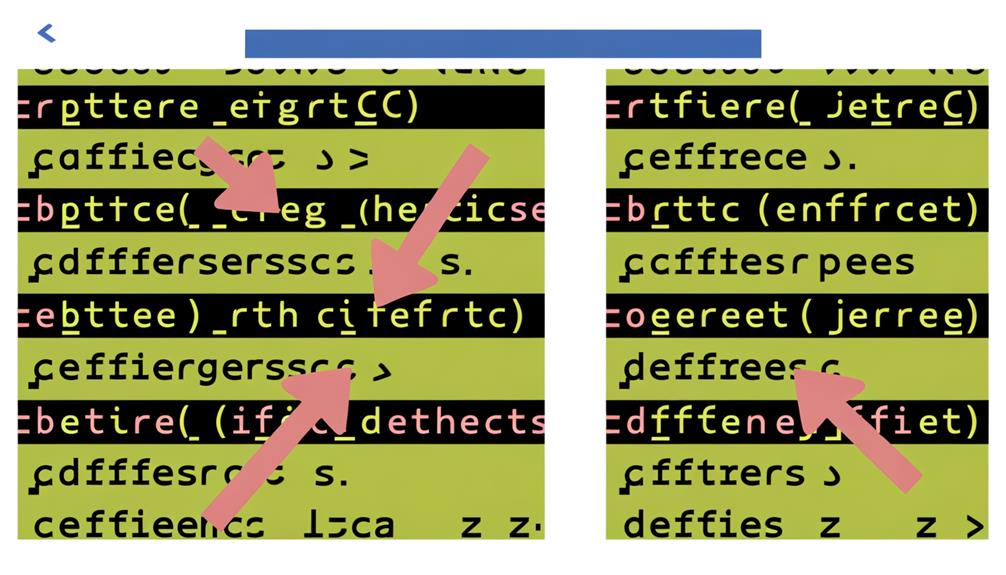
Comparing strings in C can be achieved using the strncmp() function, which allows for the precise comparison of a specified number of characters between two strings. This function is essential for tasks like sorting, searching, and filtering data based on specific criteria within strings.
It returns an integer value that indicates the relationship between the compared strings, making it a powerful tool for conditional operations. By specifying the number of characters to compare, developers can exercise fine-grained control over the comparison process, enabling accurate and efficient string evaluations.
Additionally, strncmp() is crucial for performing case-insensitive comparisons, ensuring that the comparison process isn't affected by the case of the characters.
Character Occurrence
Locating the first occurrence of a character in a string is a fundamental task in string manipulation and can be achieved using the strchr() function. This function returns a pointer to the first occurrence of a specified character in the given string. It's a powerful tool for efficiently locating specific characters within a string, enabling precise manipulation and extraction of data.
When used in combination with other string functions, such as string comparison or tokenization, it provides the foundation for complex string processing tasks.
- strchr() efficiently locates the first occurrence of a character in a string.
- It returns a pointer to the located character within the string.
- This function is essential for extracting specific information or manipulating strings based on character occurrences.
String Search
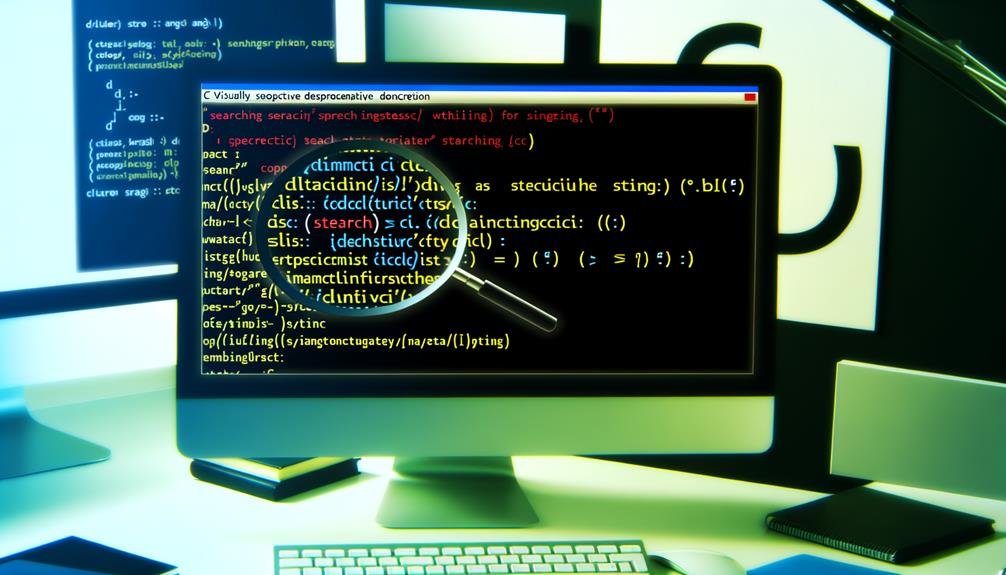
Efficiently identifying the first occurrence of a substring within a string is a fundamental aspect of string manipulation. This is facilitated by the strstr() function.
This function allows us to search for a specific substring within a larger string. It provides the index where the substring begins if it is found.
Using strstr() is liberating for programmers as it streamlines the process of locating and extracting specific patterns or substrings. This enables efficient parsing and manipulation of textual data.
By using strstr(), we can quickly and accurately identify the position of the desired substring. This empowers us to perform targeted actions based on the search results.
This function is invaluable for a wide range of use cases, from data processing to algorithmic implementations. It offers a powerful tool for string manipulation and analysis.
String Token Break
The function strtok() effectively breaks a string into a series of tokens based on a specified delimiter, facilitating the extraction of individual components for further processing or analysis.
- Enables parsing of strings into distinct components.
- Simplifies extraction of words or elements from a sentence or phrase.
- Provides a powerful tool for text processing and analysis.
Strtok() is a versatile function that empowers programmers to efficiently manipulate and extract valuable information from strings. By leveraging this function, developers can easily handle complex string manipulation tasks and streamline the processing of textual data.
Lowercase and Uppercase String
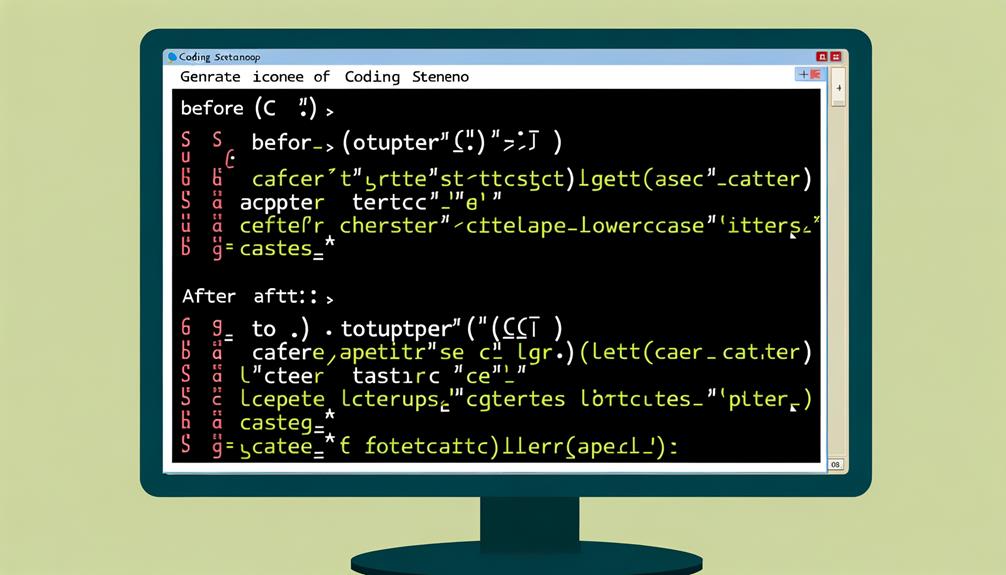
Str functions in C provide efficient methods for converting strings to lowercase and uppercase, allowing programmers to manipulate string case for various use cases.
The strlwr() function converts a string to lowercase, while the strupr() function converts a string to uppercase. These functions are beneficial for standardizing string formats, enabling case-insensitive comparisons, and facilitating independent manipulation of string case.
While strlwr() and strupr() aren't included in the Standard Library and require custom implementation, they offer valuable functionality for C programmers.
Whether it's for standardizing string case or performing case-insensitive searches, the ability to convert strings to lowercase and uppercase is a fundamental capability that enhances the versatility and practicality of C programming for string manipulation.
Custom Implementation
Moving on from discussing Lowercase and Uppercase String functions, let's now focus on the custom implementation of string functions in C, which enhances the versatility and practicality of string manipulation for C programmers.
When implementing custom string functions in C, it's essential to consider the following:
- Efficient Memory Management: Custom implementations should optimize memory allocation and deallocation for improved performance.
- Error Handling: Custom functions need to handle errors effectively to ensure robustness and reliability.
- Compatibility and Portability: Custom functions should be designed with compatibility across different platforms and compilers in mind.
Implementing custom string functions empowers C programmers to tailor their solutions to specific requirements, providing greater control and flexibility in string manipulation tasks.
Example Usage
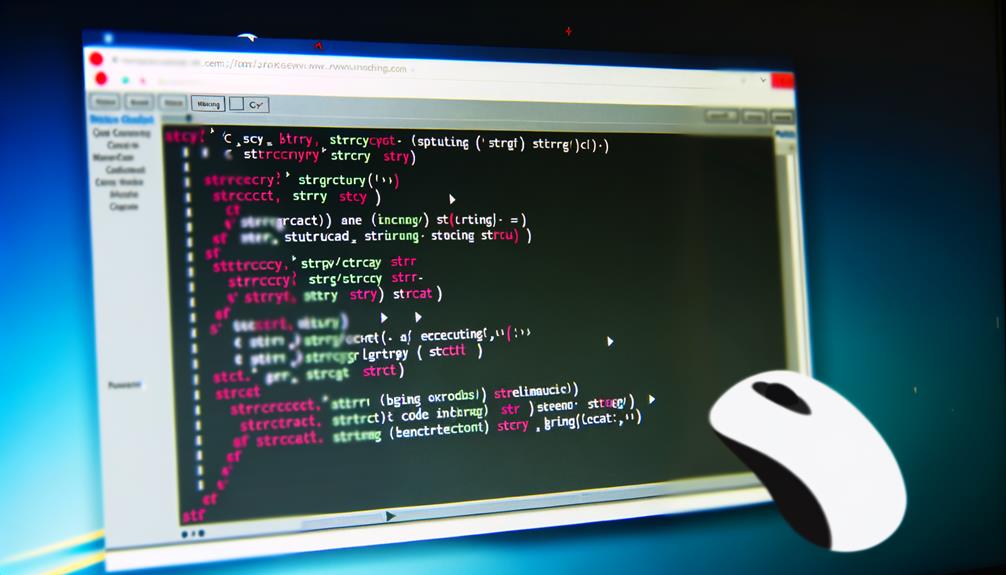
While working with string functions in C programming, it's essential to understand the practical application of each function through example usage.
For instance, to copy a specified number of characters from one string to another, we can use strncpy() like this:
strncpy(destination, source, 5).
Similarly, to concatenate a specified number of characters from the second string to the first, we can utilize strncat(), such as
strncat(str1, str2, 3).
Moreover, when comparing a specified number of characters between two strings, we can employ strncmp() as shown here:
int result = strncmp(str3, str4, 4).
These examples demonstrate how to use these string functions effectively, allowing programmers to understand their practical implementation and apply them liberally in their code.
Benefits and Use Cases
Discussing the practical application of each string function in C programming, it becomes crucial to examine the benefits and use cases they offer, allowing programmers to understand their significance and apply them effectively in various scenarios.
These essential string functions provide significant advantages and are widely used in programming.
- Useful for creating a duplicate or extracting a substring from a string.
- Useful for combining multiple strings into one.
- Useful for standardizing string formats or case-insensitive comparisons.
These functions are essential for manipulating and processing strings efficiently, enabling programmers to perform a wide range of tasks such as string manipulation, comparison, and searching.
Understanding the benefits and use cases of these functions is crucial for mastering string handling in C programming.
Frequently Asked Questions
What Are Some Common Errors or Pitfalls to Watch Out for When Using String Copy and Duplicate Functions in C?
When using string copy and duplicate functions in C, common errors or pitfalls to watch out for include:
- Buffer overflows
- Not checking for null terminators
- Memory leaks
It's essential to ensure that the destination buffer has enough space to accommodate the copied string and to always terminate the string properly.
Additionally, not freeing the memory allocated for duplicated strings can lead to memory leaks, so managing memory allocation is crucial when using these functions.
How Can String Concatenation Functions Like Strncat Be Used Efficiently to Avoid Buffer Overflow or Memory Allocation Issues?
To efficiently use string concatenation functions like strncat, we carefully determine the size of the destination buffer and ensure it has enough space to accommodate the concatenated result.
By calculating the available space and limiting the number of characters to concatenate, we can prevent buffer overflow and memory allocation issues.
This approach allows for safe and efficient string concatenation without risking data corruption or memory violations.
Are There Any Performance Differences Between Using Strncmp and Other Comparison Methods for Strings in C?
Yes, there can be performance differences between using strncmp and other comparison methods for strings in C.
This is due to the specific implementation of each function and the nature of the comparison being performed.
It's important to consider factors such as the length of the strings and the number of characters being compared, as well as any potential optimizations or inefficiencies in the underlying code.
How Can the Character Occurrence Functions Like Strchr and Strrchr Be Utilized in Practical Applications, Such as Text Processing or Data Analysis?
We can utilize the character occurrence functions like strchr and strrchr in practical applications, such as text processing or data analysis, by efficiently locating specific characters within strings.
This is useful for tasks such as extracting specific information from text data, identifying patterns or delimiters, and parsing structured data.
These functions provide essential capabilities for manipulating and analyzing textual information, enabling efficient and reliable data processing.
What Are Some Best Practices for Using String Search and Token Break Functions to Handle Complex or Multi-Dimensional Data in C Programming?
When handling complex or multi-dimensional data in C programming, it's crucial to apply best practices for string search and token break functions.
Utilize strstr() for efficient substring searches and strtok() to break strings based on delimiters.
Conclusion
In mastering these essential string functions for C programmers, we've equipped ourselves with indispensable tools for manipulating and analyzing strings effectively.
From copying and concatenating to comparing and searching, these functions enhance our proficiency in string handling.
With custom implementations for lowercase and uppercase conversions, we've expanded the versatility and adaptability of these functions.
By understanding their usage and benefits, we've strengthened our ability to process strings, making us more competent C programmers.